Difference between revisions of "Open Control Architecture"
Line 18: | Line 18: | ||
{{Block |Systems run a local MQTT broker to distribute data both internally and to enable external subscriptions. }}{{Block | The external network identifier, used for all data published to external MQTT brokers, is stored under '''''global.networkId''''' }}{{Block |In the real world, devices and networks are nested - a building may have a network ID, but this may be fed from a plantroom, also with its own identity. To accommodate nested networks, a dash is used to prefix topics (and hence the network ID) and thereby maintain a five level topic structure - important for wildcard subscriptions to work..}} | {{Block |Systems run a local MQTT broker to distribute data both internally and to enable external subscriptions. }}{{Block | The external network identifier, used for all data published to external MQTT brokers, is stored under '''''global.networkId''''' }}{{Block |In the real world, devices and networks are nested - a building may have a network ID, but this may be fed from a plantroom, also with its own identity. To accommodate nested networks, a dash is used to prefix topics (and hence the network ID) and thereby maintain a five level topic structure - important for wildcard subscriptions to work..}} | ||
myNetwork/plantroomNode/plantroomNode/ | myNetwork/plantroomNode/plantroomNode/settings/title = "Main Plantroom Controller" | ||
block1/blk1node/blk1node/ | block1/blk1node/blk1node/settings/title = "Block 1 Controller" | ||
Adding a prefix to sub-networks ensures networks remain unique on a higher level server. E.g. There may be two unconnected networks with sub-networks called "''block1"''. | Adding a prefix to sub-networks ensures networks remain unique on a higher level server. E.g. There may be two unconnected networks with sub-networks called "''block1"''. | ||
myNetwork-block1/blk1node/blk1node/ | myNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller" | ||
anotherNetwork-block1/blk1node/blk1node/ | anotherNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller" | ||
{{Block | Data from within a node or from connected devices is assigned a local network identifier of "'''''local'''''" (stored under '''''global.localNetworkId''''') so that the node's external network identifier can be changed without affecting internal software. Separate internal and external identifiers also allows for separate message rates, where local traffic can be high speed. Node-RED is used to apply '''''Reporting By Exception''''' rules, to limit traffic to significant values (e.g. 0.25C), and save bandwidth. }} | {{Block | Data from within a node or from connected devices is assigned a local network identifier of "'''''local'''''" (stored under '''''global.localNetworkId''''') so that the node's external network identifier can be changed without affecting internal software. Separate internal and external identifiers also allows for separate message rates, where local traffic can be high speed. Node-RED is used to apply '''''Reporting By Exception''''' rules, to limit traffic to significant values (e.g. 0.25C), and save bandwidth. }} | ||
Revision as of 01:47, 5 June 2022
Basic Architecture
A node can be:
- a function block within Node-RED
- an instance of Node-RED
- any point (server or device) in the communications network
Communications
myNetwork/plantroomNode/plantroomNode/settings/title = "Main Plantroom Controller"
block1/blk1node/blk1node/settings/title = "Block 1 Controller"
Adding a prefix to sub-networks ensures networks remain unique on a higher level server. E.g. There may be two unconnected networks with sub-networks called "block1".
myNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller"
anotherNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller"
Handling Data
- The Node has two outputs - one for local traffic, and one for external traffic.
- Report by exception rules are applied to external traffic.
- The Node formats topics to the five level standard, adding group and device if missing.
- The Node stores data into global.readings, along with any attributes within the incoming message, as well as timestamps.
- Additional routing can be performed within Node-RED, including selection of retained vs unretained routes.
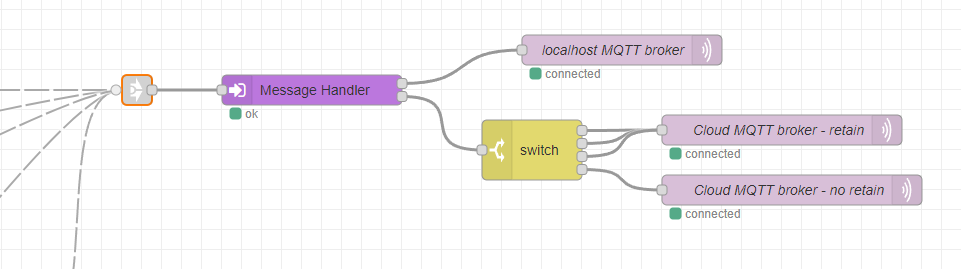
Handler Nodes use a configuration node to store setup information including network and node identities.
Data Groups
- dat (operational data)
- stat (statistics and status)
- settings (persistent settings)
- cmd (a command)
- set (command to change a setting)
- system (software and networking)
- meter (meter data)
- alarm (alarms and warnings)
- ana (analogue readings)
- json (JSON formatted data)
- modbus (Modbus serial data)
- pcdb (pcdb data)
- design (preloaded design data)
Data Attributes
- units (units for data)
- title (short description)
- points (maximum data points to store in history)
- deadband (the deadband used for external communications)
- timestamp (unix time data is generated or handled)
- changed (unix time data last changed)
- attributes (an object containing custom attributes)
- A special command cmd/sync can be sent to a nodes message handler asking it to publish a full JSON object for a device (or devices), including all data groups, values, and attributes. The command payload is an MQTT topic including wildcards, e.g. local/# (all), or +/+/boiler1/#. The reply for each device that matches is sent on the corresponding topic, e.g. myNetwork/node123/boiler1/json/sync and can be handled by a receiving system.
- Packaging a message as a JSON object, value and attributes, and send this instead of just the value. A receiving system can work out the payload is JSON and handle appropriately.
Node-RED Global Objects
The current data can be viewed in the data explorer.
Settings have a type, may have maximum and minimum values, units and a title. Certain types require additional fields such as select from a list settings.
The snapshot below shows a variety of setting types including numeric/text values, html, or JSON objects and arrays.
e.g. a message with a topic myNetwork/node123/myDevice/dat/outputTemperature would be stored in (global).readings.myNetwork.myDevice.dat.outputTemperature.value
Internally, message topics can be truncated and the network and node will be filled in. The data group will be assumed to be "dat" if not supplied, representing operational data.
e.g. a message with a topic setpoint would be stored in (global).readings.local.myNode.dat.setpoint.value
The following code could be used to read data and its attributes for output.
var localNetworkId = global.get("localNetworkId"); var node = global.get("node"); var reading = global.get("readings." + localNetworkId + "." + node + ".dat.outputTemperature"); var output = "The value of " + reading.title + " is " + reading.value + reading.units; // "The value of Output Temperature is 35°C