Difference between revisions of "Open Control Architecture"
Line 36: | Line 36: | ||
{{Block | Each instance of Node-RED has an identifier, stored under '''''global.node''''' }} | {{Block | Each instance of Node-RED has an identifier, stored under '''''global.node''''' }} | ||
{{Block |'''camelCase''' is used for variable names and topics, where the first letter of additional words are capitalised, and spaces removed. | {{Block |'''camelCase''' is used for variable names and topics, where the first letter of additional words are capitalised, and spaces removed. |
Revision as of 13:52, 5 June 2022
Basic Architecture
- Real-time global commutation (<0.5s latency) of any payload type between millions of devices
- Open source and licence free for life
- Battle hardened, standard and secure technologies
- Simple to use for plumbers and electricians
- Can connect to anything, physical or web based, and make use of commercial services and APIs
- Implement a standard dictionary structure to enable interoperability between systems
We have chosen to base the Open Controls Architecture on Node-RED for a number of reasons:
- Open source and free to use at will
- Cross platform, from PCs, to cloud servers to embedded devices, the Raspberry Pi, and many industrial PLCs
- Very stable, having been actively developed for over 10 years with the likes of IBM
- Simple to use with a visual interface that compares to electrical wiring
- Software can be copied and pasted from one system to another, dropped in and wired up
- Infinitely versatile with standard and custom components available from an online library to achieve almost any function or connect to any system
- Complex software can be built in a hurry, dragging components in and wiring up, then refined and packaged into neat and updateable components
- Built on Node.js and JavaScript, possible the world's most active software environment as used on all web pages
- Used by almost everyone - from hobbyists through to mobile phone companies - with considerable community support
- Provides a means to easily implement new and existing technologies and online services, including machine learning, peer-to-peer communications, databases, and encryption
A node can be:
- a function block within Node-RED (a Node-RED Node)
- an instance of Node-RED (a Controller Node)
- any point in the communications network (e.g. an MQTT Broker)
Only letters, numbers, or dashes (-) should ever be used in naming.
Communications
See https://github.com/heatweb/heat-network
This must be unique (long) without spaces, and in camelCase.
myNetwork/plantroomNode/plantroomNode/settings/title = "Main Plantroom Controller"
block1/blk1node/blk1node/settings/title = "Block 1 Controller" !!! DO NOT USE COMMON NETWORK IDs
Adding a prefix to sub-networks ensures networks remain unique on a higher level server. E.g. There may be two unconnected networks with sub-networks called "block1".
myNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller"
anotherNetwork-block1/blk1node/blk1node/settings/title = "Block 1 Controller"
e.g. Ea5lQXfF2tmBlzYk
Nodes should also be given unique identities. Nodes Id's can be shorter as they only need to be unique within a network, rather than globally (in the whole world).
e.g. jxt15EwkThe titles of networks, as well as nodes, can be saved and found under its device setting.
Ea5lQXfF2tmBlzYk/jxt15Ewk/network/settings/title = "My Heat Network"
Ea5lQXfF2tmBlzYk/jxt15Ewk/jxt15Ewk/settings/title = "Main Plantroom Controller"
And to simplify for sub-nodes it is possible to use a node's id as the node's network identifier.
a5lQXfF2tmBlzYk-fg4jS8s7/fg4jS8s7/network/settings/title = "Block 1 Heat Network"
a5lQXfF2tmBlzYk-fg4jS8s7/fg4jS8s7/fg4jS8s7/settings/title = "Block 1 Controller"
a5lQXfF2tmBlzYk-fg4jS8s7-nnf555dE/nnf555dE/nnf555dE/settings/title = "Block 1, Flat 17 Controller"
Handling Data
- The Node has two outputs - one for local traffic, and one for external traffic.
- Report by exception rules are applied to external traffic.
- The Node formats topics to the five level standard, adding group and device if missing.
- The Node stores data into global.readings, along with any attributes within the incoming message, as well as timestamps.
- Additional routing can be performed within Node-RED, including selection of retained vs unretained routes.
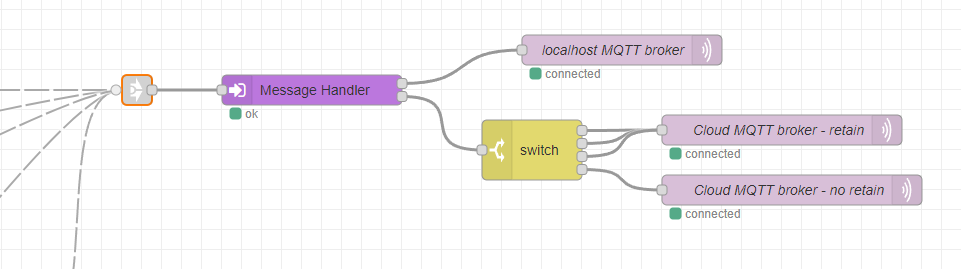
Handler Nodes use a configuration node to store setup information including network and node identities.
Data Groups
- dat (operational data)
- stat (statistics and status)
- settings (persistent settings)
- cmd (a command)
- set (command to change a setting)
- system (software and networking)
- meter (meter data)
- alarm (alarms and warnings)
- ana (analogue readings)
- json (JSON formatted data)
- modbus (Modbus serial data)
- design (preloaded design data)
- TIL (Thermal Integration Ltd.)
- PCDB (SAP Product Characteristics Database)
- BENCHMARK (Benchmark information)
Manufacturer specific data groups can be used by systems to load a manufacturer's default data attributes, enabling the protocol to be extended as required.
They allow, for example, multiple organisations to publish the same data on a device (such as a heat network) without collisions.
Data Attributes
- units (units for data)
- title (short description)
- points (maximum data points to store in history)
- deadband (the deadband used for external communications)
- timestamp (unix time data is generated or handled)
- changed (unix time data last changed)
- attributes (an object containing custom attributes)
The flow below shows a message object, with attributes, before and after going through an MQTT connector.
Note that only the topic and payload survive transit.
Note that dat/tOut is a standard topic, so the attributes (units and title) would be added back in by a Handler Node.
- A special command cmd/sync can be sent to a nodes message handler asking it to publish a full JSON object for a device (or devices), including all data groups, values, and attributes. The command payload is an MQTT topic including wildcards, e.g. local/# (all), or +/+/boiler1/#. The reply for each device that matches is sent on the corresponding topic, e.g. myNetwork/node123/boiler1/json/sync and can be handled by a receiving system.
- Packaging a message as a JSON object, value and attributes, and send this instead of just the value. A receiving system can work out the payload is JSON and handle appropriately.
Node-RED Global Objects
The current data can be viewed in the data explorer.
Settings have a type, may have maximum and minimum values, units and a title. Certain types require additional fields such as select from a list settings.
The snapshot below shows a variety of setting types including numeric/text values, html, or JSON objects and arrays.
e.g. a message with a topic myNetwork/node123/myDevice/dat/outputTemperature would be stored in (global).readings.myNetwork.myDevice.dat.outputTemperature.value
Internally, message topics can be truncated and the network and node will be filled in. The data group will be assumed to be "dat" if not supplied, representing operational data.
e.g. a message with a topic setpoint would be stored in (global).readings.local.myNode.dat.setpoint.value
The following code could be used to read data and its attributes for output.
var localNetworkId = global.get("localNetworkId"); var node = global.get("node"); var reading = global.get("readings." + localNetworkId + "." + node + ".dat.outputTemperature"); var output = "The value of " + reading.title + " is " + reading.value + reading.units; // "The value of Output Temperature is 35°C